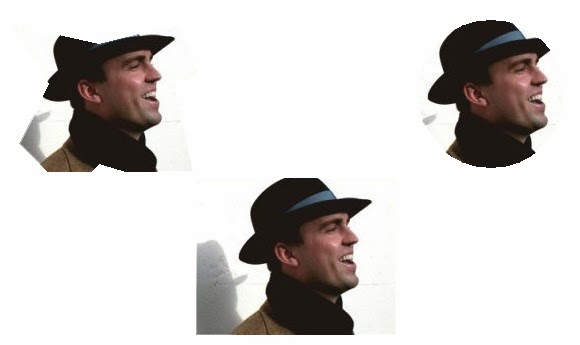
First we need a canvas tag
<canvas id="c" width="200" height="158"></canvas>
attempt to get the canvas and its drawing context in JavaScript.
/
/
Grab
the
Canvas
and
Drawing Context
var canvas
=
document
.getElementById
(
'c'
)
;
var ctx
=
canvas.getContext
(
'
2
d'
)
;
Drawing the Image into the Canvas
// Create an image element
var img = document.createElement('IMG');
// When the image is loaded, draw it
img.onload = function () {
ctx.drawImage(img, 0, 0);
}
// Specify the src to load the image
img.src = "http://i.imgur.com/gwlPu.jpg";
Clip that Image
// Create an image element
var img = document.createElement('IMG');
// When the image is loaded, draw it
img.onload = function () {
// Save the state, so we can undo the clipping
ctx.save();
// Create a circle
ctx.beginPath();
ctx.arc(106, 77, 74, 0, Math.PI * 2, false);
// Clip to the current path
ctx.clip();
ctx.drawImage(img, 0, 0);
// Undo the clipping
ctx.restore();
}
// Specify the src to load the image
img.src = "http://i.imgur.com/gwlPu.jpg";
You can clip to any shape you want. You just need to define your path.
// Create an image element
var img = document.createElement('IMG');
// When the image is loaded, draw it
img.onload = function () {
// Save the state, so we can undo the clipping
ctx.save();
// Create a shape, of some sort
ctx.beginPath();
ctx.moveTo(10, 10);
ctx.lineTo(100, 30);
ctx.lineTo(180, 10);
ctx.lineTo(200, 60);
ctx.arcTo(180, 70, 120, 0, 10);
ctx.lineTo(200, 180);
ctx.lineTo(100, 150);
ctx.lineTo(70, 180);
ctx.lineTo(20, 130);
ctx.lineTo(50, 70);
ctx.closePath();
// Clip to the current path
ctx.clip();
ctx.drawImage(img, 0, 0);
// Undo the clipping
ctx.restore();
}
// Specify the src to load the image
img.src = "http://i.imgur.com/gwlPu.jpg";
Thanku...........
Sign up here with your email
ConversionConversion EmoticonEmoticon